R307 Optical Fingerprint Scanner Library for Arduino – Documentation
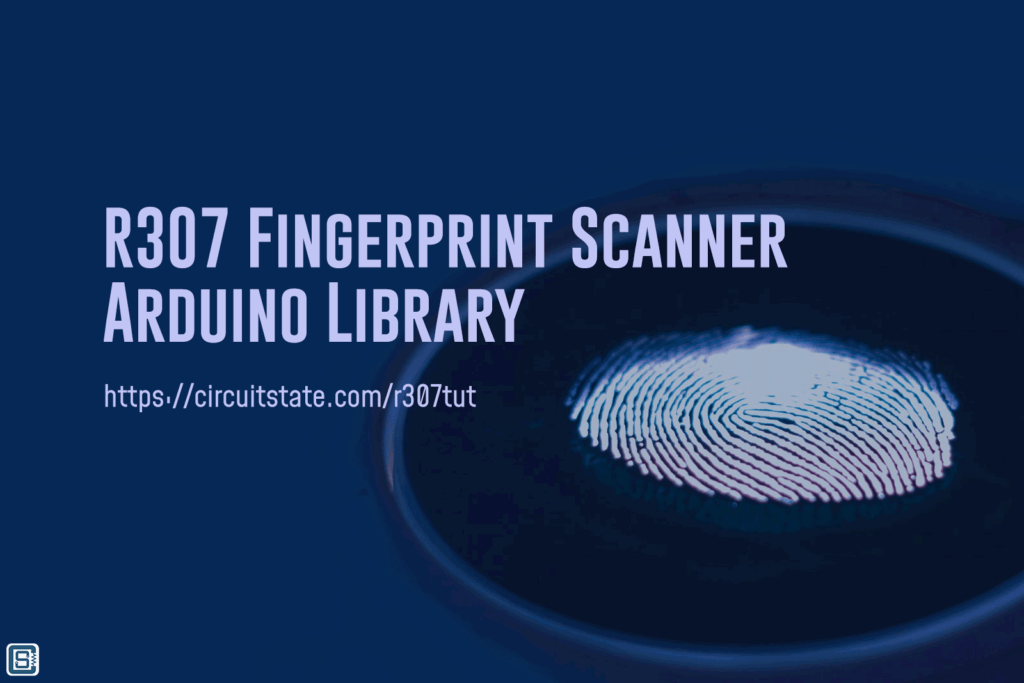
This is the documentation for R307 fingerprint scanner Arduino library developed by Vishnu Mohanan. A tutorial on interfacing R307 scanners with Arduino compatible boards is available on a separate page.
The most widely used library for the R30X series modules is the Adafruit Fingerprint Sensor library. It was released 7 years ago, it is hard to understand due to lack of documentation and has limited features. That’s when I decided to write one myself. Writing libraries has become an exercise for me.
Since the instruction set and confirmation codes are compatible with many versions of the fingerprint scanner, you could easily modify this library to interface a different type. The code is easy to understand and is shared as an open source project. The library is not complete yet. Many functions yet to be implemented. But the basic enrolling, searching and matching functions work. All that is working are demonstrated in the example Arduino sketch.
The library consists of a header file R30X_Fingerprint.h and a CPP file R30X_Fingerprint.cpp. The header file will give an overview of the library and it includes all instructions codes, default values, class declarations, parameters and functions declarations.
Since the module uses UART to communicate, you have the choice of choosing the serial port you want. With boards like Arduino Uno with single UART, you can use the SoftwareSerial for interfacing the fingerprint module and hardware serial for debugging. Debugging is optional. Currently SoftwareSerial
is used for AVR and ESP8266 boards. So when initializing, you must create a SoftwareSerial
object and send it to the constructor function. I tested the module with Arduino Due and used the hardware serial.
- Library Details
- Installation
- Dependencies
- Constants
- Classes
- Member Variables
- Member Functions
- begin()
- resetParameters()
- verifyPassword()
- setPassword()
- setAddress()
- setBaudrate()
- reinitializePort()
- setSecurityLevel()
- setDataLength()
- portControl()
- sendPacket()
- receivePacket()
- readSysPara()
- captureAndRangeSearch()
- captureAndFullSearch()
- generateImage()
- downloadImage()
- generateCharacter()
- generateTemplate()
- downloadCharacter()
- uploadCharacter()
- saveTemplate()
- loadTemplate()
- deleteTemplate()
- clearLibrary()
- matchTemplates()
- searchLibrary()
- getTemplateCount()
- Example Sketch
- Tested Boards
- Troubleshooting
- GitHub
- Downloads
- Links
Library Details
- Latest version: v1.3.1
- Author: Vishnu Mohanan
- Source: https://github.com/vishnumaiea/R30X-Fingerprint-Sensor-Library
- Documentation: https://circuitstate.com/r307doc
- Tutorial: https://circuitstate.com/r307tut
- Initial release: IST 07:35 PM, 08-04-2019, Monday
- License: MIT
Installation
The library is now part of the official Arduino library set. To install it on your computer, open the Library Manager from the Arduino IDE and search for “R30X fingerprint scanner”. Then install the latest version from the list.
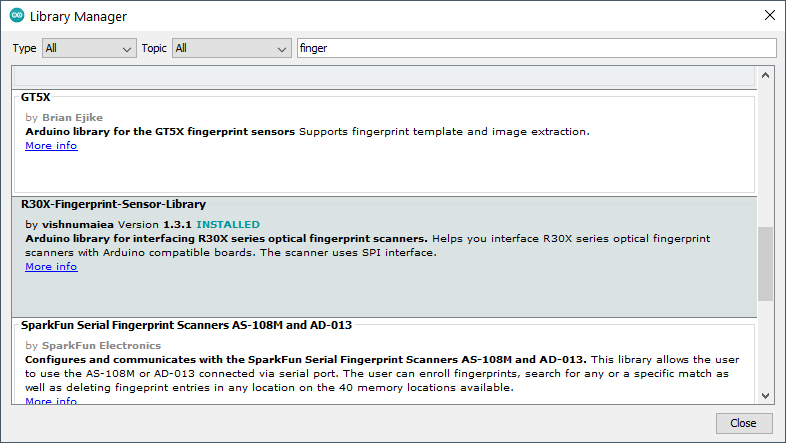
Another way is to download the library as a ZIP from GitHub and extract the contents to the libraries folder inside your Arduino sketches folder.
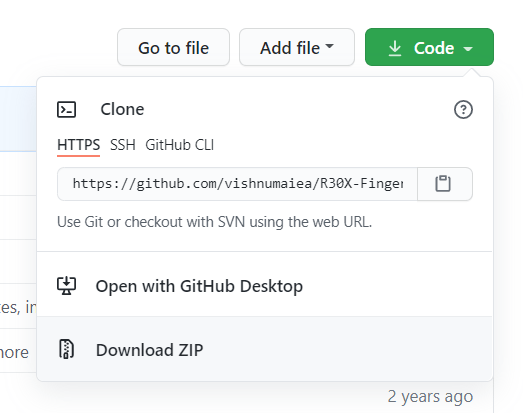
Dependencies
The SoftwareSerial header is required if your board does not have additional hardware UARTs.
Arduino.h
SoftwareSerial.h
Constants
All the constants are defined inside the main header file. It includes all the commands, response codes and default values. FPS_DEBUG
is a macro to enable or disable the display of debug information. Comment out the line if you do not want debug information to be printed. The serial port to which the debug info is sent is set by the debugPort
macro. The default one is Serial
, the first/default serial port on the board.
//=========================================================================//
#define FPS_DEBUG //uncomment this line to enable debug info to be printed
#define debugPort Serial //the serisl port to which debug info will be sent
//=========================================================================//
//Response codes from FPS to the commands sent to it
//FPS = Fingerprint Scanner
#define FPS_RESP_OK 0x00U //command executed successfully
#define FPS_RESP_RECIEVEERR 0x01U //packet receive error
#define FPS_RESP_NOFINGER 0x02U //no finger detected
#define FPS_RESP_ENROLLFAIL 0x03U //failed to enroll the finger
#define FPS_RESP_OVERDISORDERFAIL 0x04U //failed to generate character file due to over-disorderly fingerprint image
#define FPS_RESP_OVERWETFAIL 0x05U //failed to generate character file due to over-wet fingerprint image
#define FPS_RESP_OVERDISORDERFAIL2 0x06U //failed to generate character file due to over-disorderly fingerprint image
#define FPS_RESP_FEATUREFAIL 0x07U //failed to generate character file due to over-wet fingerprint image
#define FPS_RESP_DONOTMATCH 0x08U //fingers do not match
#define FPS_RESP_NOTFOUND 0x09U //no valid match found
#define FPS_RESP_ENROLLMISMATCH 0x0AU //failed to combine character files (two character files (images) are used to create a template)
#define FPS_RESP_BADLOCATION 0x0BU //addressing PageID is beyond the finger library
#define FPS_RESP_INVALIDTEMPLATE 0x0CU //error when reading template from library or the template is invalid
#define FPS_RESP_TEMPLATEUPLOADFAIL 0x0DU //error when uploading template
#define FPS_RESP_PACKETACCEPTFAIL 0x0EU //module can not accept more packets
#define FPS_RESP_IMAGEUPLOADFAIL 0x0FU //error when uploading image
#define FPS_RESP_TEMPLATEDELETEFAIL 0x10U //error when deleting template
#define FPS_RESP_DBCLEARFAIL 0x11U //failed to clear fingerprint library
#define FPS_RESP_WRONGPASSOWRD 0x13U //wrong password
#define FPS_RESP_IMAGEGENERATEFAIL 0x15U //fail to generate the image due to lackness of valid primary image
#define FPS_RESP_FLASHWRITEERR 0x18U //error when writing flash
#define FPS_RESP_NODEFINITIONERR 0x19U //no definition error
#define FPS_RESP_INVALIDREG 0x1AU //invalid register number
#define FPS_RESP_INCORRECTCONFIG 0x1BU //incorrect configuration of register
#define FPS_RESP_WRONGNOTEPADPAGE 0x1CU //wrong notepad page number
#define FPS_RESP_COMPORTERR 0x1DU //failed to operate the communication port
#define FPS_RESP_INVALIDREG 0x1AU //invalid register number
#define FPS_RESP_SECONDSCANNOFINGER 0x41U //secondary fingerprint scan failed due to no finger
#define FPS_RESP_SECONDENROLLFAIL 0x42U //failed to enroll second fingerprint
#define FPS_RESP_SECONDFEATUREFAIL 0x43U //failed to generate character file due to lack of enough features
#define FPS_RESP_SECONDOVERDISORDERFAIL 0x44U //failed to generate character file due to over-disorderliness
#define FPS_RESP_DUPLICATEFINGERPRINT 0x45U //duplicate fingerprint
//-------------------------------------------------------------------------//
//Received packet verification status codes from host device
#define FPS_RX_OK 0x00U //when the response is correct
#define FPS_RX_BADPACKET 0x01U //if the packet received from FPS is badly formatted
#define FPS_RX_WRONG_RESPONSE 0x02U //unexpected response
#define FPS_RX_TIMEOUT 0x03U //when no response was received
//-------------------------------------------------------------------------//
//Packet IDs
#define FPS_ID_STARTCODE 0xEF01U
#define FPS_ID_STARTCODEHIGH 0xEFU
#define FPS_ID_STARTCODELOW 0x01U
#define FPS_ID_COMMANDPACKET 0x01U
#define FPS_ID_DATAPACKET 0x02U
#define FPS_ID_ACKPACKET 0x07U
#define FPS_ID_ENDDATAPACKET 0x08U
//-------------------------------------------------------------------------//
//Command codes
#define FPS_CMD_SCANFINGER 0x01U //scans the finger and collect finger image
#define FPS_CMD_IMAGETOCHARACTER 0x02U //generate char file from a single image and store it to one of the buffers
#define FPS_CMD_MATCHTEMPLATES 0x03U //match two fingerprints precisely
#define FPS_CMD_SEARCHLIBRARY 0x04U //search the fingerprint library
#define FPS_CMD_GENERATETEMPLATE 0x05U //combine both character buffers and generate a template
#define FPS_CMD_STORETEMPLATE 0x06U //store the template on one of the buffers to flash memory
#define FPS_CMD_LOADTEMPLATE 0x07U //load a template from flash memory to one of the buffers
#define FPS_CMD_EXPORTTEMPLATE 0x08U //export a template file from buffer to computer
#define FPS_CMD_IMPORTTEMPLATE 0x09U //import a template file from computer to sensor buffer
#define FPS_CMD_EXPORTIMAGE 0x0AU //export fingerprint image from buffer to computer
#define FPS_CMD_IMPORTIMAGE 0x0BU //import an image from computer to sensor buffer
#define FPS_CMD_DELETETEMPLATE 0x0CU //delete a template from flash memory
#define FPS_CMD_CLEARLIBRARY 0x0DU //clear fingerprint library
#define FPS_CMD_SETSYSPARA 0x0EU //set system configuration register
#define FPS_CMD_READSYSPARA 0x0FU //read system configuration register
#define FPS_CMD_SETPASSWORD 0x12U //set device password
#define FPS_CMD_VERIFYPASSWORD 0x13U //verify device password
#define FPS_CMD_GETRANDOMCODE 0x14U //get random code from device
#define FPS_CMD_SETDEVICEADDRESS 0x15U //set 4 byte device address
#define FPS_CMD_PORTCONTROL 0x17U //enable or disable comm port
#define FPS_CMD_WRITENOTEPAD 0x18U //write to device notepad
#define FPS_CMD_READNOTEPAD 0x19U //read from device notepad
#define FPS_CMD_HISPEEDSEARCH 0x1BU //highspeed search of fingerprint
#define FPS_CMD_TEMPLATECOUNT 0x1DU //read total template count
#define FPS_CMD_SCANANDRANGESEARCH 0x32U //read total template count
#define FPS_CMD_SCANANDFULLSEARCH 0x34U //read total template count
#define FPS_DEFAULT_TIMEOUT 2000 //UART reading timeout in milliseconds
#define FPS_DEFAULT_BAUDRATE 57600 //9600*6
#define FPS_DEFAULT_RX_DATA_LENGTH 64 //the max length of data in a received packet
#define FPS_DEFAULT_SECURITY_LEVEL 3 //the threshold at which the fingerprints will be matched
#define FPS_DEFAULT_SERIAL_BUFFER_LENGTH 300 //length of the buffer used to read the serial data
#define FPS_DEFAULT_PASSWORD 0xFFFFFFFF
#define FPS_DEFAULT_ADDRESS 0xFFFFFFFF
#define FPS_BAD_VALUE 0x1FU //some bad value or paramter was delivered
//=========================================================================//
Classes
The main class with variables and functions.
R30X_FPS
Member Variables
These are public and private member variables. Some parameters have both array and whole bit versions such as devicePasswordL
which is a 32-bit value, and devicePassword[4]
which is an array of four 8-bit values. This is just done for convenience, and must not confuse you. When a packet is created and extracted, different set of variables are used, prefixed by rx
and tx
. Some values are variable in length and therefore pointers are used, example *txDataBuffer
. mySerial
is a pointer to a Stream
object which is used to communicate with different interfaces. swSerial
is a pointer only used for SoftwareSerial
interface and hwSerial
is only used for HardwareSerial
interface. They will be conditionally included by the compiler depending on the platform you’re compiling for.
//common parameters
public:
uint16_t startCodeL; //packet start marker
uint8_t startCode[2]; //packet start marker
uint32_t devicePasswordL; //32-bit single value version of password (L = long)
uint32_t deviceAddressL; //module's address
uint8_t devicePassword[4]; //array version of password
uint8_t deviceAddress[4]; //device address as an array
uint16_t statusRegister; //contents of the FPS status register
uint16_t systemID; //fixed value 0x0009
uint16_t librarySize; //library memory size
uint16_t securityLevel; //threshold level for fingerprint matching
uint16_t dataPacketLengthCode;
uint16_t dataPacketLength; //the max length of data in packet. can be 32, 64, 128 or 256
uint16_t baudMultiplier; //value between 1-12
uint32_t deviceBaudrate; //UART speed (9600 * baud multiplier)
//transmit packet parameters
uint8_t txPacketType; //type of packet
uint16_t txPacketLengthL; //length of packet (Data + Checksum)
uint8_t txInstructionCode; //instruction to be sent to FPS
uint16_t txPacketChecksumL; //checksum long value
uint8_t txPacketLength[2]; //packet length as an array
uint8_t *txDataBuffer; //packet data buffer
uint16_t txDataBufferLength; //length of actual data in a packet
uint8_t txPacketChecksum[2]; //packet checksum as an array
//receive packet parameters
uint8_t rxPacketType; //type of packet
uint16_t rxPacketLengthL; //packet length long
uint8_t rxConfirmationCode; //the return codes from the FPS
uint16_t rxPacketChecksumL; //packet checksum long
uint8_t rxPacketLength[2]; //packet length as an array
uint8_t *rxDataBuffer; //packet data buffer
uint32_t rxDataBufferLength; //the length of the data only. this doesn't include instruction or confirmation code
uint8_t rxPacketChecksum[2]; //packet checksum as array
uint16_t fingerId; //location of fingerprint in the library
uint16_t matchScore; //the match score of comparison of two fingerprints
uint16_t templateCount; //total number of fingerprint templates in the library
private:
Stream *mySerial; //stream class is used to facilitate communication
SoftwareSerial *swSerial; //for those devices with only one hardware UART
HardwareSerial *hwSerial; //for those devices with multiple hardware UARTs
Member Functions
Not all of these functions are fully implemented. All that working are demonstrated in the example Arduino sketch.
R30X_FPS(HardwareSerial *hs, uint32_t password = FPS_DEFAULT_PASSWORD, uint32_t address = FPS_DEFAULT_ADDRESS);
void begin(uint32_t baud); //initializes the communication port
void resetParameters(void); //initialize and reset and all parameters
uint8_t verifyPassword(uint32_t password = FPS_DEFAULT_PASSWORD); //verify the user supplied password
uint8_t setPassword(uint32_t password); //set FPS password
uint8_t setAddress(uint32_t address = FPS_DEFAULT_ADDRESS); //set FPS address
uint8_t setBaudrate(uint32_t baud); //set UART baudrate, default is 57000
uint8_t reinitializePort(uint32_t baud);
uint8_t setSecurityLevel(uint8_t level); //set the threshold for fingerprint matching
uint8_t setDataLength(uint16_t length); //set the max length of data in a packet
uint8_t portControl(uint8_t value); //turn the comm port on or off
uint8_t sendPacket(uint8_t type, uint8_t command, uint8_t *data = NULL, uint16_t dataLength = 0); //assemble and send packets to FPS
uint8_t receivePacket(uint32_t timeout = FPS_DEFAULT_TIMEOUT); //receive packet from FPS
uint8_t readSysPara(void); //read FPS system configuration
uint8_t captureAndRangeSearch(uint16_t captureTimeout, uint16_t startId, uint16_t count); //scan a finger and search a range of locations
uint8_t captureAndFullSearch(void); //scan a finger and search the entire library
uint8_t generateImage(void); //scan a finger, generate an image and store it in the buffer
uint8_t exportImage(void); //export a fingerprint image from the sensor to the computer
uint8_t importImage(uint8_t *dataBuffer); //import a fingerprint image from the computer to sensor
uint8_t generateCharacter(uint8_t bufferId); //generate character file from image
uint8_t generateTemplate(void); //combine the two character files and generate a single template
uint8_t exportCharacter(uint8_t bufferId); //export a character file from the sensor to computer
uint8_t importCharacter(uint8_t bufferId, uint8_t *dataBuffer); //import a character file to the sensor from computer
uint8_t saveTemplate(uint8_t bufferId, uint16_t location); //store the template in the buffer to a location in the library
uint8_t loadTemplate(uint8_t bufferId, uint16_t location); //load a template from library to one of the buffers
uint8_t deleteTemplate(uint16_t startLocation, uint16_t count); //delete a set of templates from library
uint8_t clearLibrary(void); //delete all templates from library
uint8_t matchTemplates(void); //match the templates stored in the two character buffers
uint8_t searchLibrary(uint8_t bufferId, uint16_t startLocation, uint16_t count); //search the library for a template stored in the buffer
uint8_t getTemplateCount(void); //get the total no. of templates in the library
Functions are explained below.
R30X_Fingerprint (HardwareSerial *hs, uint32_t password = FPS_DEFAULT_PASSWORD, uint32_t address = FPS_DEFAULT_ADDRESS);
R30X_Fingerprint (SoftwareSerial *ss, uint32_t password = FPS_DEFAULT_PASSWORD, uint32_t address = FPS_DEFAULT_ADDRESS);
These are the constructors for hardware and software serial interfaces. These are not overloaded but conditionally selected. The usage is shown in the example sketch. First parameter is the serial port you want to use to communicate to the module, which is different from debug port. Next you have to send the 32-bit password and address. If you want to use the default ones, send nothing.
begin()
void begin (uint32_t baud);
This initializes the serial port with specified baud rate.
resetParameters()
void resetParameters (void);
Resets all parameters to default values.
verifyPassword()
uint8_t verifyPassword (uint32_t password = FPS_DEFAULT_PASSWORD);
Verifies the given password. You need to verify the password before doing anything. Otherwise the module will always respond with a 0x21
error code. If you want to test for the default password, pass nothing. Otherwise send your custom password. Returns the confirmation code.
setPassword()
uint8_t setPassword (uint32_t password);
This updates the password on the module. The password is also saved to the parameter variables. Returns the confirmation code.
setAddress()
uint8_t setAddress (uint32_t address = FPS_DEFAULT_ADDRESS);
Updates the device address. Once you successfully change it, all further data packets must include the new address. Returns the confirmation code.
setBaudrate()
uint8_t setBaudrate (uint32_t baud);
This modifies the baudrate at which your module is communicating. It first changes the baudrate multiplier on the module, closes the current serial port and reinitializes the serial port with the new baudrate. This can be difficult at times. Returns the confirmation code.
reinitializePort()
uint8_t reinitializePort (uint32_t baud);
Reinitializes the port with a new baud rate.
setSecurityLevel()
uint8_t setSecurityLevel (uint8_t level);
Sets the the security level. Values can be 1-5. Returns the confirmation code.
setDataLength()
uint8_t setDataLength (uint16_t length);
Sets the data length. Values can be 32, 64, 128, 256 bytes. Returns the confirmation code.
portControl()
uint8_t portControl (uint8_t value);
Turns the communication port on or off from the module side. Value can be 1 = ON or 0 = OFF. Returns the confirmation code.
sendPacket()
uint8_t sendPacket (uint8_t type, uint8_t command, uint8_t* data = NULL, uint16_t dataLength = 0);
Creates a packet with command and data, and sends it out to the module. When there’s not data accompanied with a command, you can leave the data
and dataLength
parameters empty. If you enable debugging, the packet will be printed to the debug port. Returns the confirmation code.
receivePacket()
uint8_t receivePacket (uint32_t timeout=FPS_DEFAULT_TIMEOUT);
Reads data from the serial monitor, extracts parameters from the packet and saves them to the variables. You could optionally send the timeout the host must wait for a reply. If you leave this empty, FPS_DEFAULT_TIMEOUT
will be used which is 2 seconds. Returns the confirmation code.
readSysPara()
vuint8_t readSysPara (void);
Reads the contents of the System Configuration Register (16 bytes) and saves values to the variables. Returns the confirmation code.
captureAndRangeSearch()
uint8_t captureAndRangeSearch (uint16_t captureTimeout, uint16_t startId, uint16_t count);
Scans a finger and search between a range of locations in the fingerprint library. You should send the timeout for the scan, start location and the number of locations to search. If the operation was successful, the results are stored in fingerId
and matchScore
. Returns the confirmation code.
captureAndFullSearch()
uint8_t captureAndFullSearch (void);
Scans a finger and search the entire fingerprint library for a match. There’s no control over the timeout or anything. The module does everything itself. If you want to specify the timeout, use the captureAndRangeSearch()
and use the entire range for searching. If the operation was successful, the results are stored in fingerId
and matchScore
. Returns the confirmation code.
generateImage()
uint8_t generateImage (void);
Scans the finger once and store the captured image to the Image Buffer. You may read the content of the buffer after executing this. Returns the confirmation code.
downloadImage()
uint8_t downloadImage (void);
This retrieves the content of the Image Buffer to the host. After the confirmation from the module side, it will start transferring of data. The host must be prepared to accept the data. Returns the confirmation code. This function is not fully implemented.
generateCharacter()
uint8_t generateCharacter (uint8_t bufferId);
Generates a character file from the image stored in the Image Buffer. The generated character file is saved to one of the two character buffers, which must be specified to the function. Returns the confirmation code.
generateTemplate()
uint8_t generateTemplate (void);
Generates a template file combining the two character file buffers. Therefore two scans must be performed in order to generate a template file. The generated template file will be stored to the CharBuffer1
. Returns the confirmation code.
downloadCharacter()
uint8_t downloadCharacter (uint8_t bufferId);
Retrieves the character file content from one of the two buffers. You should specify the buffer number which can be 1 or 2. After the confirmation packet, the module will start sending the data. The host must be prepared to accept the data. Returns the confirmation code. This function is not fully implemented.
uploadCharacter()
uint8_t uploadCharacter (uint8_t bufferId, uint8_t* dataBuffer);
This allows you to send a character file you already have to one of the buffers inside the module. This will allow you to save and restore fingerprint database. You should send the buffer number and data. After the confirmation the host must send the data to the module. Returns the confirmation code. This function is not fully implemented.
saveTemplate()
uint8_t saveTemplate (uint8_t bufferId, uint16_t location);
This function saves the template file in one of the buffers to any location in the fingerprint library. You should specify the buffer ID and the location you want save to. Returns the confirmation code.
loadTemplate()
uint8_t loadTemplate (uint8_t bufferId, uint16_t location);
Loads one of the character buffers with a template from the fingerprint library. You should specify the buffer you want to use and the location in the fingerprint library you want the template to load from. Returns the confirmation code.
deleteTemplate()
uint8_t deleteTemplate (uint16_t startLocation, uint16_t count);
Deletes one or more templates from the specified range in the fingerprint library. Returns the confirmation code.
clearLibrary()
uint8_t clearLibrary (void);
Erases all contents of the fingerprint library. This action is irreversible. Returns the confirmation code.
matchTemplates()
uint8_t matchTemplates (void);
Precisely matches the contents of the two character file buffers. The result is stored in the matchScore
variable. Returns the confirmation code.
searchLibrary()
uint8_t searchLibrary (uint8_t bufferId, uint16_t startLocation, uint16_t count);
Searches the fingerprint library for a template stored in one of the character buffers. You should specify the buffer number, start location and number of templates to match. The results are stored in fingerId
and matchScore
. Returns the confirmation code.
getTemplateCount()
uint8_t getTemplateCount (void);
Gets the total number of templates available in the fingerprint library. The result is saved on templateCount
. Returns the confirmation code.
Example Sketch
The example Arduino sketch to test the fingerprint scanner is available here – https://github.com/vishnumaiea/R30X-Fingerprint-Sensor-Library/blob/master/examples/R30X-FPS-Test/R30X-FPS-Test.ino
I wrote this code for Arduino Due which has 4 hardware serial ports. I am using first serial port Serial
for debugging and Serial1
for fingerprint scanner interface. The password and address are the default 0xFFFFFFFF
. Three of these parameters are passed to the constructor. You must use the password and address of your module if they were ever changed.
In the setup()
function, we first initialize the debugging port and the fingerprint module. fps
is the object we’re using. Then we have to verify the password before doing anything else. Otherwise the scanner will refuse to execute our commands. Optionally you may set a new address, or verify the existing address.
In the loop()
function, we periodically check the serial port for incoming data. When data is available, it is read as a string and it is checked for valid commands and parameters. If it’s a valid command, rest of the parameters are extracted from the string in order as firstParam
, secondParam
and thirdParam
. Then the parameters are sent to corresponding function to execute. Once the command is executed, the results are stored in the variables and we wait for new instructions. Following is the list of the available commands you can send through the serial monitor. No line ending characters should be appended when sending commands via serial monitor. This can be done by setting line ending to No line ending in Arduino serial monitor.
clrlib
– clear librarytmpcnt
– get templates countreadsys
– read system parameterssetdatlen <data length>
– set data lengthcapranser <timeout> <start location> <quantity>
– capture and range search library for fingerprintcapfulser
– capture and full search the library for fingerprintenroll <location>
– enroll new fingerprintverpwd <password>
– verify 4 byte device passwordsetpwd <password>
– set new 4 byte device passwordsetaddr <address>
– set new 4 byte device addresssetbaud <baudrate>
– set the baudratereinitprt <baudrate>
– reinitialize the port without changing device configurationsetseclvl <level>
– set security levelgenimg
– generate imagegenchar <buffer id>
– generate character file from imagegentmp
– generate template from character bufferssavtmp <buffer id> <location>
– save template to library from bufferlodtmp <buffer id> <location>
– load template from library to bufferdeltmp <start location> <quantity>
– delete one or more templates from librarymattmp
– precisely match two templates available on buffersserlib <buffer id> <start location> <quantity>
– search library for content on the buffer
What appears between < and > are parameters that must be sent with the command (< or > should not be included). Commands and parameters must be separated by a single whitespace. For example, “enroll 12” will enroll a new fingerprint at location #12.
The enrollFinger()
function in the example sketch implements the fingerprint enrolling process.
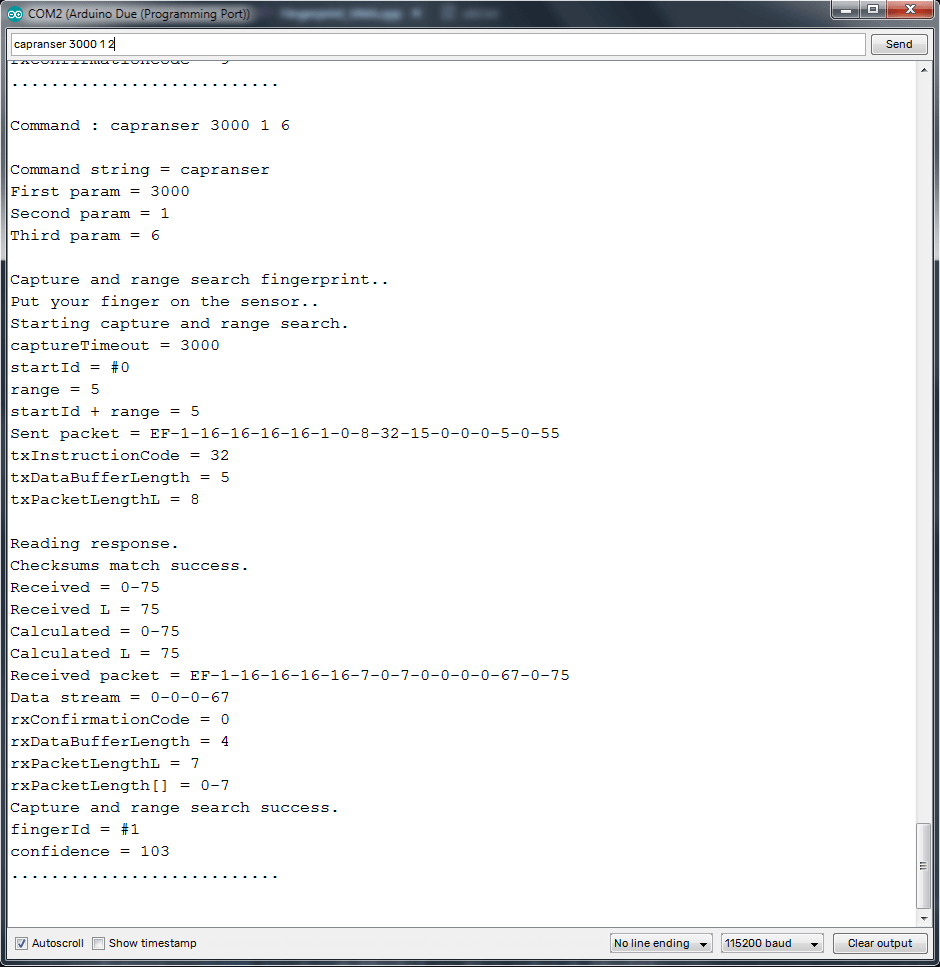
If you found any error with documentation or code, however small it is, feel free to tell me about it. Your questions are always welcome.
Tested Boards
The library was tested with Arduino Due and Arduino Uno using R307 fingerprint scanner. To wire up, connect the TX and RX pins to the TX1 and RX1 pins of Due or Mega. If you’re using Uno or similar boards with only one hardware UART, use SoftwareSerial for the fingerprint sensor and hardware UART for debugging.
Even though not tested, the library is expected to work with other Arduino compatible microcontrollers and boards such as ESP8266, ESP32, STM32 Nucleo, TI Launchpad etc.
Troubleshooting
When something is not working, upload the example sketch to your board and run the commands to check if they’re working as expected. Some of the issues you may encounter are,
- Getting “Password is not correct” message
New modules will be coming with the default password and device address 0xFFFFFFFF. If the example sketch complains about wrong password, then try running the setpwd command. For example,setpwd FFFFFFFF
- Getting “Invalid command” message
If your serial terminal application is sending NL/CR characters automatically, try turning this off. For example, you can turn this feature off at Arduino serial monitor by selecting No line ending.
GitHub
Downloads
- SYNO Demo [ZIP]
- SFG Demo [RAR]
- R30X Fingerprint Scanner User Manual [PDF]
- R300 Fingerprint Scanner User Manual [PDF]
- R301T Fingerprint Scanner User Manual [PDF]
- R302 Fingerprint Scanner User Manual [PDF]
- R303 Fingerprint Scanner User Manual [PDF]
- R303T Fingerprint Scanner User Manual [PDF]
- R305 Fingerprint Scanner User Manual [PDF]
- R306 Fingerprint Scanner User Manual [PDF]
- R307 Fingerprint Scanner User Manual [PDF]
- R308 Fingerprint Scanner User Manual [PDF]
- R311 Fingerprint Scanner User Manual [PDF]
Links
Short Link
- Short URL to this page – https://circuitstate.com/r307doc